Guest Blog Post: Week Two - Day Two Work Experience (2014)
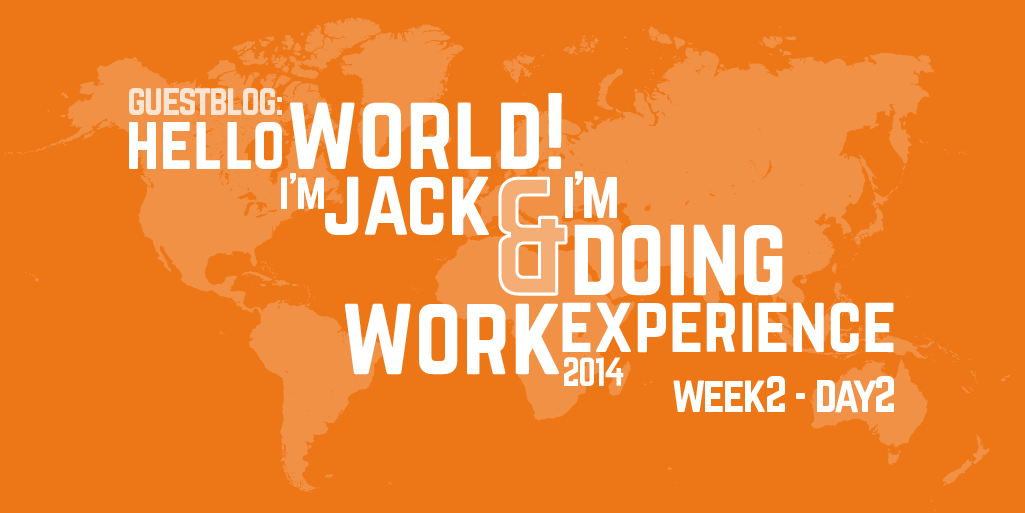
In his second week of work experience at endjin, Jack, 15, writes a console application to display TeamCity build statuses using a BusyLight.
————— Today we had a very productive day. Richard and I created a console application where we were able to retrieve the success or failure of a TeamCity build, and make a Busylight change colour to show the status. TeamCity is a 'continuous integration platform' which lets you manage all your code in one place. When someone publishes changes to some code, TeamCity tries to build and test the code to make sure it's all still working.
As with our previous project which made the Busylight change colour, we included the Busylight SDK in the project. We also included the TeamCitySharp library, which is a free package for accessing TeamCity via their REST API.
We checked for build success, failure or an error by providing the build configuration ID from the TeamCity website and getting back information about the build using TeamCitySharp's Build and BuildStatus types.
We made it so a failed build made the light flash red and pink with a period of just pink. Whereas a solid green light indicated that the TeamCity build had succeeded without any problems. We also made it so if there was an error it would turn solid pink.
private void UpdateLight()
{
var buildids = teamcitydataservice.GetBuildIds("BuildConfigIds.txt");
var lastBuilds = teamcitydataservice.GetTeamCityBuilds(buildids);
if (lastBuilds.All(build => build.Status == BuildStatus.SUCCESS.ToString()))
{
lightController.Light(BusylightColor.Green);
}
else if (lastBuilds.Any(build => build.Status == BuildStatus.FAILURE.ToString()))
{
this.FlashLightWhenError();
}
else if (lastBuilds.Any(build => build.Status == BuildStatus.ERROR.ToString()))
{
this.GetPinkBusyLightColor();
}
}
We made a field for users to enter their TeamCity account and details.
static void Main(string[] args)
{
var server = GetServerFromUser();
var client = new TeamCityClient(server);
var username = GetUsernameFromUser();
var password = GetPasswordFromUser();
client.Connect(username,password);
After this we made it so that the when you put in your details the password would show as asterisks to protect your security.
private static string GetPasswordFromUser()
{
string pass = "";
Console.Write("Enter your password: ");
ConsoleKeyInfo key;
do
{
key = Console.ReadKey(true);
if (key.Key != ConsoleKey.Backspace && key.Key != ConsoleKey.Enter)
{
pass += key.KeyChar;
Console.Write("*");
}
else
{
if (key.Key == ConsoleKey.Backspace && pass.Length > 0)
{
pass = pass.Substring(0, (pass.Length - 1));
Console.Write("\b \b");
}
}
}
while (key.Key != ConsoleKey.Enter);
return pass;
}
We also made sure that Richard's details were not still in the code when we uploaded it to GitHub to protect his security.
Finally we "resharped" (Note from editor, this means used ReSharper but I quite like this version!) a number of areas so the code looked neater, and I learnt to refactor the code out into classes.
namespace TeamCityLightNotifierService
{
class Program
{
static void Main(string[] args)
{
var lightnotifier = new LightNotifier();
lightnotifier.UpdateLightContinuously();
}
}
}
It was great to see when it worked!
You can find the application on GitHub.